Basics JavaScript stuff
JavaScript is a cross-platform, object-oriented scripting language. The v8 by Chrome runs the Node and make JS be a performatic language.
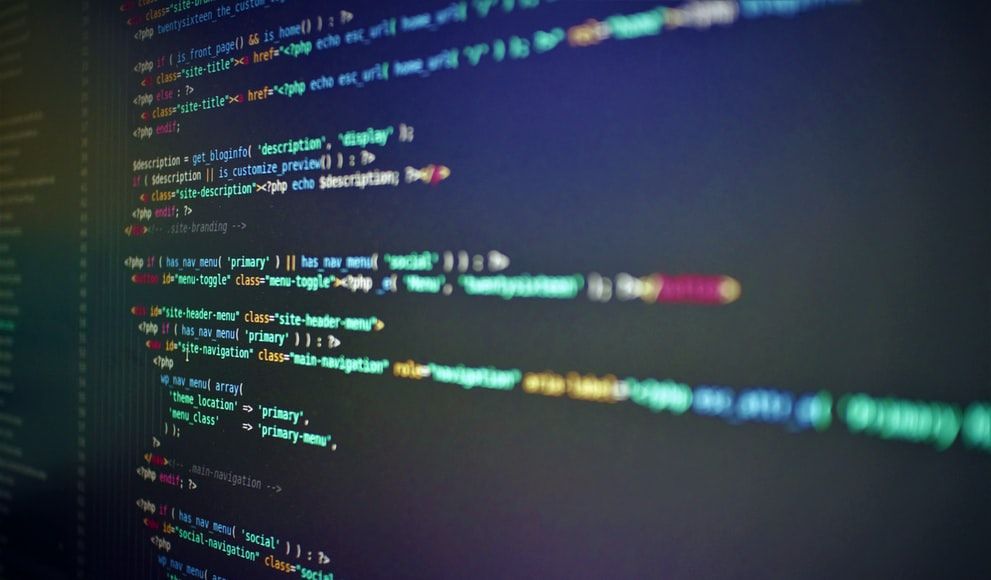
Por Hugo | | JavaScript
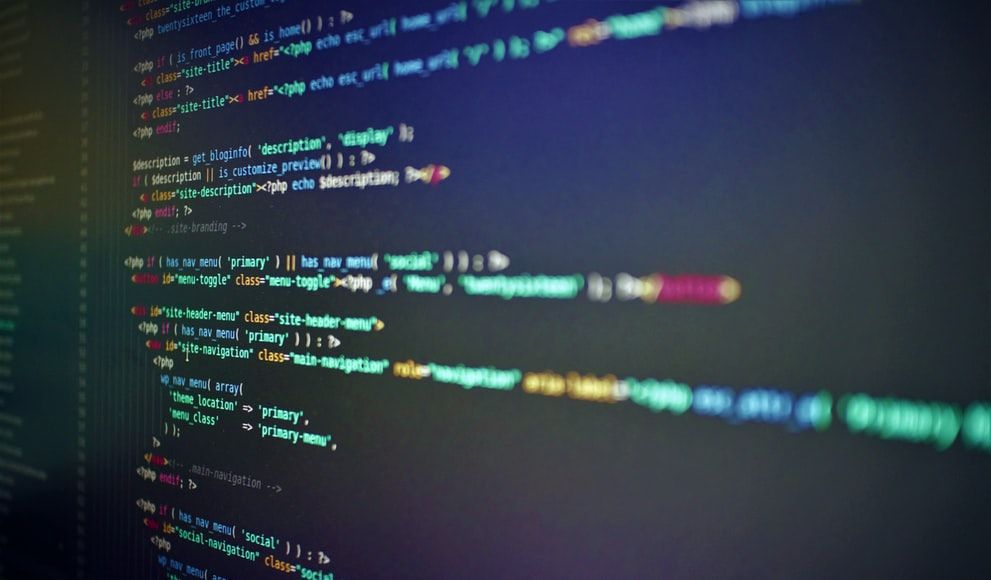
Introduction
JavaScript is a cross-platform, object-oriented scripting language. It is a small and lightweight language. Inside a host environment (for example, a web browser), JavaScript can be connected to the objects of its environment to provide programmatic control over them.
JavaScript contains a standard library of objects, such as Array, Date, and Math, and a core set of language elements such as operators, control structures, and statements. Core JavaScript can be extended for a variety of purposes by supplementing it with additional objects; for example:

JavaScript and Java
JavaScript and Java are similar in some ways but fundamentally different in some others. The JavaScript language resembles Java but does not have Java's static typing and strong type checking.
JavaScript follows most Java expression syntax, naming conventions and basic control-flow constructs which was the reason why it was renamed from LiveScript to JavaScript. In contrast to Java's compile-time system of classes built by declarations, JavaScript supports a runtime system based on a small number of data types representing numeric, Boolean, and string values. JavaScript has a prototype-based object model instead of the more common class-based object model.
The prototype-based model provides dynamic inheritance; that is, what is inherited can vary for individual objects. JavaScript also supports functions without any special declarative requirements. Functions can be properties of objects, executing as loosely typed methods. JavaScript is a very free-form language compared to Java. You do not have to declare all variables, classes, and methods. You do not have to be concerned with whether methods are public, private, or protected, and you do not have to implement interfaces. Variables, parameters, and function return types are not explicitly typed.
Right Text
Functions can be properties of objects, executing as loosely typed methods. JavaScript is a very free-form language compared to Java. You do not have to declare all variables, classes, and methods. You do not have to be concerned with whether methods are public, private, or protected, and you do not have to implement interfaces. Variables, parameters, and function return types are not explicitly typed.
Left Text
Functions can be properties of objects, executing as loosely typed methods. JavaScript is a very free-form language compared to Java. You do not have to declare all variables, classes, and methods. You do not have to be concerned with whether methods are public, private, or protected, and you do not have to implement interfaces. Variables, parameters, and function return types are not explicitly typed.
A bulleted list
- stuff
- stuff
- stuff
- stuff
Lázaro está citando isso pra editar um bloco de citação depois rsrs - Lázaro.
Hello world
To get started with writing JavaScript, open the Scratchpad and write your first "Hello world" JavaScript code:
console.log("Hello World")
With Functions
function sayHelloWorld() {
return 'Hello World';
}
You can build a collection of items
- a collection of fruits
let fruits = ["Apple", "Orange","Pinapple"]
- use objects inside array itens
let ArrayOfObjects = [
{ name: 'Sr.Nothing', age: 14 },
{ name: 'Sr.OtherGuy', age: 25 },
];
- Numbers are valid too
let array = [1,2,3,4,5,6,7,8,9,10]
Bibliotecas e Frameworks
Front-end | Back-end | Mobile | titutlo | titutlo |
React | Node.js | React Native | info | info |
Vue | Express | React Native | info | info |
Angular | Nest.js | React Native | info | info |
Another Example
Em bloco de codigo
var fruit = ['cherries', 'apples', 'bananas'];
fruit.sort(); // ['apples', 'bananas', 'cherries']
var scores = [1, 10, 2, 21];
scores.sort(); // [1, 10, 2, 21]
// Observe que 10 vem antes do 2,
// porque '10' vem antes de '2' em ponto de código Unicode.
var things = ['word', 'Word', '1 Word', '2 Words'];
things.sort(); // ['1 Word', '2 Words', 'Word', 'word']
// Em Unicode, números vêem antes de letras maiúsculas,
// as quais vêem antes das minúsculas.